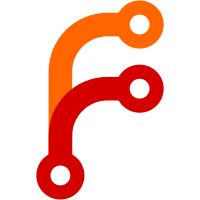
* Port previous ethernet scheme * Add ipd * Fix initfs rebuilds, use QEMU user networking addresses in ipd * Add tcp/udp, netutils, dns, and network config * Add fsync to network driver * Add dns, router, subnet by default * Fix e1000 driver. Make ethernet and IP non-blocking to avoid deadlocks * Add orbital server, WIP * Add futex * Add orbutils and orbital * Update libstd, orbutils, and orbital Move ANSI key encoding to vesad * Add orbital assets * Update orbital * Update to add login manager * Add blocking primitives, block for most things except waitpid, update orbital * Wait in waitpid and IRQ, improvements for other waits * Fevent in root scheme * WIP: Switch to using fevent * Reorganize * Event based e1000d driver * Superuser-only access to some network schemes, display, and disk * Superuser root and irq schemes * Fix orbital
34 lines
732 B
Rust
34 lines
732 B
Rust
use collections::BTreeMap;
|
|
use spin::Mutex;
|
|
|
|
use sync::WaitCondition;
|
|
|
|
#[derive(Debug)]
|
|
pub struct WaitMap<K, V> {
|
|
inner: Mutex<BTreeMap<K, V>>,
|
|
condition: WaitCondition
|
|
}
|
|
|
|
impl<K, V> WaitMap<K, V> where K: Ord {
|
|
pub fn new() -> WaitMap<K, V> {
|
|
WaitMap {
|
|
inner: Mutex::new(BTreeMap::new()),
|
|
condition: WaitCondition::new()
|
|
}
|
|
}
|
|
|
|
pub fn send(&self, key: K, value: V) {
|
|
self.inner.lock().insert(key, value);
|
|
self.condition.notify();
|
|
}
|
|
|
|
pub fn receive(&self, key: &K) -> V {
|
|
loop {
|
|
if let Some(value) = self.inner.lock().remove(key) {
|
|
return value;
|
|
}
|
|
self.condition.wait();
|
|
}
|
|
}
|
|
}
|