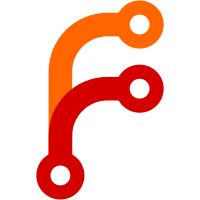
* Port previous ethernet scheme * Add ipd * Fix initfs rebuilds, use QEMU user networking addresses in ipd * Add tcp/udp, netutils, dns, and network config * Add fsync to network driver * Add dns, router, subnet by default * Fix e1000 driver. Make ethernet and IP non-blocking to avoid deadlocks * Add orbital server, WIP * Add futex * Add orbutils and orbital * Update libstd, orbutils, and orbital Move ANSI key encoding to vesad * Add orbital assets * Update orbital * Update to add login manager * Add blocking primitives, block for most things except waitpid, update orbital * Wait in waitpid and IRQ, improvements for other waits * Fevent in root scheme * WIP: Switch to using fevent * Reorganize * Event based e1000d driver * Superuser-only access to some network schemes, display, and disk * Superuser root and irq schemes * Fix orbital
63 lines
1.6 KiB
Rust
63 lines
1.6 KiB
Rust
#![feature(allocator)]
|
|
#![feature(const_fn)]
|
|
|
|
#![allocator]
|
|
#![no_std]
|
|
|
|
use spin::Mutex;
|
|
use linked_list_allocator::Heap;
|
|
|
|
extern crate spin;
|
|
extern crate linked_list_allocator;
|
|
|
|
static HEAP: Mutex<Option<Heap>> = Mutex::new(None);
|
|
|
|
pub unsafe fn init(offset: usize, size: usize) {
|
|
*HEAP.lock() = Some(Heap::new(offset, size));
|
|
}
|
|
|
|
#[no_mangle]
|
|
pub extern fn __rust_allocate(size: usize, align: usize) -> *mut u8 {
|
|
if let Some(ref mut heap) = *HEAP.lock() {
|
|
heap.allocate_first_fit(size, align).expect("out of memory")
|
|
} else {
|
|
panic!("__rust_allocate: heap not initialized");
|
|
}
|
|
}
|
|
|
|
#[no_mangle]
|
|
pub extern fn __rust_deallocate(ptr: *mut u8, size: usize, align: usize) {
|
|
if let Some(ref mut heap) = *HEAP.lock() {
|
|
unsafe { heap.deallocate(ptr, size, align) };
|
|
} else {
|
|
panic!("__rust_deallocate: heap not initialized");
|
|
}
|
|
}
|
|
|
|
#[no_mangle]
|
|
pub extern fn __rust_usable_size(size: usize, _align: usize) -> usize {
|
|
size
|
|
}
|
|
|
|
#[no_mangle]
|
|
pub extern fn __rust_reallocate_inplace(_ptr: *mut u8, size: usize,
|
|
_new_size: usize, _align: usize) -> usize
|
|
{
|
|
size
|
|
}
|
|
|
|
#[no_mangle]
|
|
pub extern fn __rust_reallocate(ptr: *mut u8, size: usize, new_size: usize,
|
|
align: usize) -> *mut u8 {
|
|
use core::{ptr, cmp};
|
|
|
|
// from: https://github.com/rust-lang/rust/blob/
|
|
// c66d2380a810c9a2b3dbb4f93a830b101ee49cc2/
|
|
// src/liballoc_system/lib.rs#L98-L101
|
|
|
|
let new_ptr = __rust_allocate(new_size, align);
|
|
unsafe { ptr::copy(ptr, new_ptr, cmp::min(size, new_size)) };
|
|
__rust_deallocate(ptr, size, align);
|
|
new_ptr
|
|
}
|